Variables, Constants and Operators
Variables
A variable is a location in memory that holds one or more values. It has a label or name to identify it and its values can be changed as the program runs.
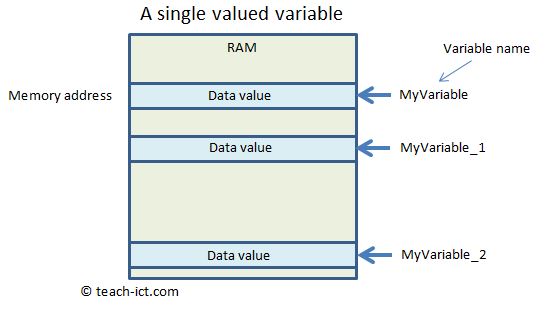
It is good practice in programming to give variables a sensible name that will help explain its purpose. What you should not do is put spaces into a name even if the language allows it, as it can be confusing and it is too easy to mistype it later
When name variable good practice is to add a underscore to separate the words in the name. For example
first_name = "Laura"
second_name = "Bradshaw"
Constants
A constant is a location in memory that holds a value. It has a label or name to identify it and its value cannot be changed as the program runs. A constant is often declared in capital letters to highlight the fact that it is not a variable.
Constant CHRISTMAS_DAY = "25th December"
Operators
Assignement Operators
Assignment operators assign values.
The simplest assignment operator is “=”. It copies the value of its right argument into the argument on its left.
MyVariable = 2
This assigns the value 2 to the variable named ‘MyVariable’.
The assignment operator can also copy a value from one variable to another. For example
MyVariable = AnotherVariable
Arithmetic Operators
You are already familiar with many simple arithmetic operations: addition, subtraction, division and multiplication. The symbols used to represent these operations in a program are mostly the same ones you see in a maths textbook:-
+ for addition
– for subtraction
* for multiplication
/ for division
Boolean Operators
boolean operator carries out a logical operation on its arguments.
Examples of boolean operators include:
AND – returns TRUE only if both arguments are true |
OR – returns TRUE if either arguments are true |
NOT – return the inverse of the boolean argument |
XOR – Exclusive OR. Returns true if either argument is true but not both. |
Boolean operators return True or False.